Build Mechanism
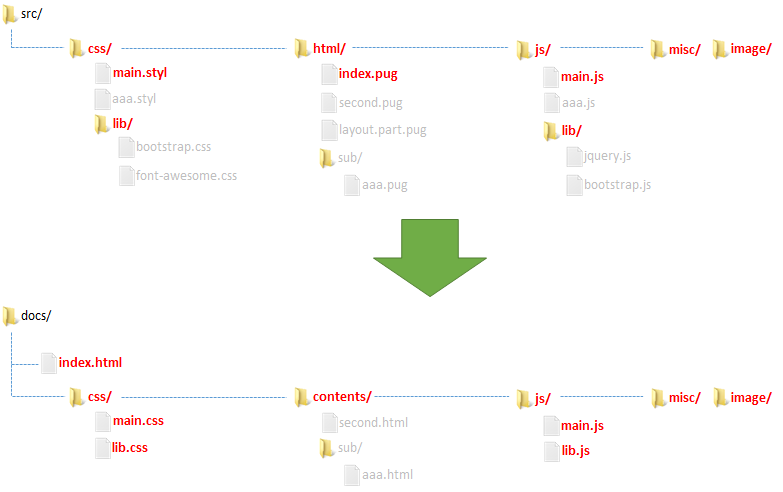
Red bold folders/files ... Fixed name folders/files required for build
Grayed thin folders/files ... User defined folders/files optional for build
The picture above depicts how source files are built into output folder.
Only red bold folders/files are recognized as build target. Thin grayed folders/files
will be ignored if they are not referred to by the red bold source files.
src/
and docs/
are customizable via --src/--dst options.
For all available options on build, please check out Usage
Build process varies depending on content type such as html/css/javascript/others.
If you want to go deep as to how SSSG works, please check the source hosted in
GitHub.
Building Stylus for CSS
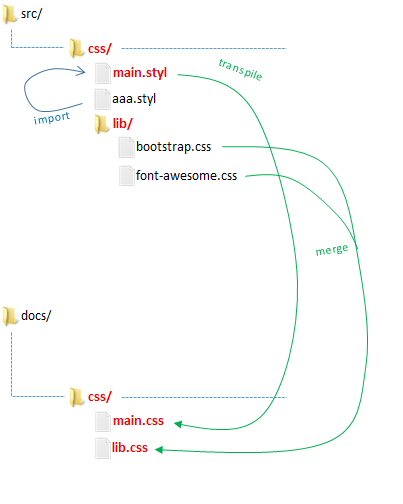
SSSG build src/css/main.styl
into docs/css/main.css
.
All library files in src/css/lib/
, in the above example,
bootstrap.css
and font-awesome.css
will be just merged into
docs/css/lib.css
.
You can modularize stylus files by utilizing @import
statement. In the example above,
main.styl
imports aaa.styl
. You can break a file into pieces based on
pages.
All files except for main.styl
and the lib files will be ignored
unless they are @import
ed by main.styl
.
In sssg serve
command, build frequencies of main.css
and lib.css
should be noted carefully.
While main.css
is dynamically and frequently updated as you edit source stylus files,
lib.css
is only compiled/updated once sssg serve
launches.
So in case you want to add library files, you need to restart sssg serve
.
Building ES6 Javascript for Browser compatible ES5 Javascript
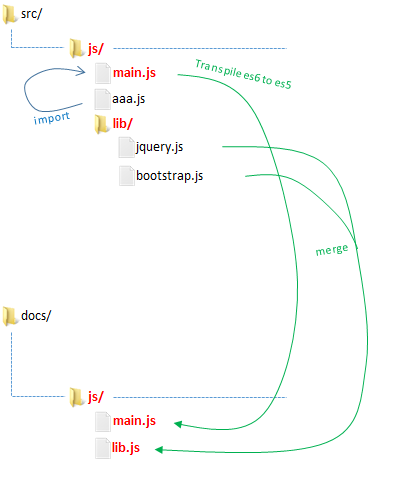
Building javascript is very similar to building css. Other than file extension and folder name, I can say they are almost the same.
SSSG build src/js/main.js
into docs/js/main.js
.
Although file extension does not change, its content transforms from es6 into
es5, which most modern(~2017) browsers support.
All library files in src/js/lib/
, in the above example,
jquery.js
and bootstrap.js
will be just merged into
docs/js/lib.js
.
.babelrc
SSSG is equipped with babel.
You can put your original .babelrc just under src/js/
so that you use any babel presets/plugins you want.
By .babelrc, for example you can write code in ReactJS(JSX) syntax.
Example for ReactJS
Move to work folder
$ cd [some empty folder]
Install ReactJS(react, react-dom) and babel presets.
$ npm init -y
$ npm install --save-dev react react-dom
$ npm install --save-dev babel-preset-react babel-preset-env
Initialize SSSG
$ sssg init
Create src/js/.babelrc
activating react plugins
{ "presets": ["react", "env"] }
From here you can write ReactJS code. If you want code samples, Check THIS out.
import from node_modues
import
statement works against global/local npm modules.
If you installed SSSG as a local npm module, you can import
modules
in node_modules
folder.
Though importing from npm_modules is helpful, it is not recommended because it imports
npm module into main.js
, not lib.js
.
As well as building stylus, in sssg serve
command, main.js
is dynamically updated and
lib.js
is just compiled once.
It is recommended to put static library files into src/js/lib/
folder to avoid
building overhead.
incremental build in sssg serve
SSSG adopts watchify
, which only compiles modified javascript files
in dependency tree.
As unmodified files are cached and not re-compiled at build time, building speed becomes
incredibly fast!
Building Pug for HTML
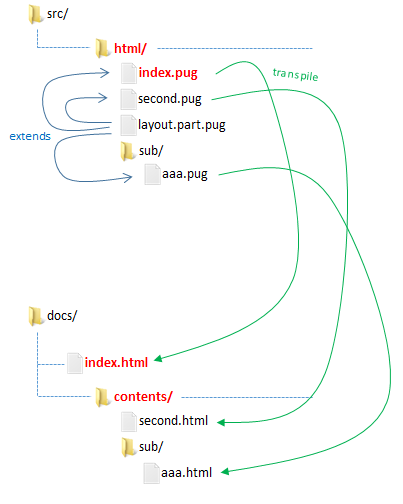
Building pug is different to previous ones.
For building stylus and es6, only main.styl
/main.js
or library files are compiled.
For pug, all pug files except for partial pug files under src/html
folder will be compiled.
Please note that src/html/index.pug
and other pug files are build in different process.
Detailed reason about this is described in source code.
partial pug files
A pug file is recognized as partial if :
- its filename ends with ".part.pug" (i.e. layout.part.pug)
- its filename starts with "_" (i.e. _layout.pug)
- its parent folder name starts with "_" (i.e. _common/layout.pug)
These partial pug files will be ignored unless they are extended
or
included
by normal pug files.
You can use this to write sharable html components or store pug variables/mixins.
src/html/index.pug
It is compiled into docs/index.html
by default.
The location of index.html
can be specified via --root|-r
option.
You should be careful to specify local resource(js/css/image) path in the pug file.
When you write
<script src="...">
or <link href="...">
or
<img src="...">
or so, ALWAYS specify its path relative to
the actual path where index.html
will be compiled into.
other .pug files
Unlike the index.pug
, all .pug files except for partial pug files will be compiled into
docs/contents/
.
Folders you create under src/html/
will be preserved and created under the
docs/contents
folder.
Like the index.pug
, local resource path should be carefully specified.
Resource path should be relative to the path where there .pug files will be compiled into.
incremental build in sssg serve
Building html goes incremental, meaning only modified file and files referencing the modified file
will be compiled.
How this is achieved is bit tricky. First, when you edit any pug file in src/html
folder,
all html file in output folder will be deleted.
After this deletion completes, http server in SSSG will send a signal to your browser to reload current page.
When your html request comes to http server by reloading signal, there are no html files yet
because they are all deleted.
Now here is a trick. Http server in SSSG tries to compile only a pug file corresponding to requested html
and returns it to you.
This greatly improves building speed for responsive previewing. In general, building time get longer as
pug files grows. If there are tens of pug files, building time would be over 10 seconds.
But with this build mechanism, building time would be 10 - 100 times faster because html files not requested
yet won't be compiled until you requests it.
Don't forget to rebuild after developing by sssg serve
has done.
Since build mechanism for html/pug is bit abnormal, please take a deep breath before publishing your
files generated in docs/
.
As html files in docs/contents, docs/index.html
might be deleted by last edit,
you should always sssg rebuild
before porting files in docs/
to anywhere.
This is not so comfortable I know. It's trade-off with build performance. I think developing speed matters
than making an effort not to forget sssg rebuild
.
Building Image/Misc files
Image files in src/image/
and misc files in src/misc/
will be
just copied into docs/image
and docs/misc
.